Shutdown
About
"Shutdown" is a twin-stick shooter game with three exciting levels where your mission is to defuse a bomb. As you fight against waves of enemies, your goal is to clear obstacles and find the bomb before time runs out.
During the project, I served as the SCRUM master for six weeks out of the eight. Our team included two developers (myself and Nils Hanssens) and three skilled artists: Stijn Bakker, Louisa Burfitt, and Tim Slappendel. Together, we collaborated to create this fun and engaging game.
Project Info
- Role: Game Programmer
- Team Size: 5
- Project Duration: 8 Weeks
- Engine: Unity C#
- Completion Date: 30th of June 2023
My contributions
Procedural Animations
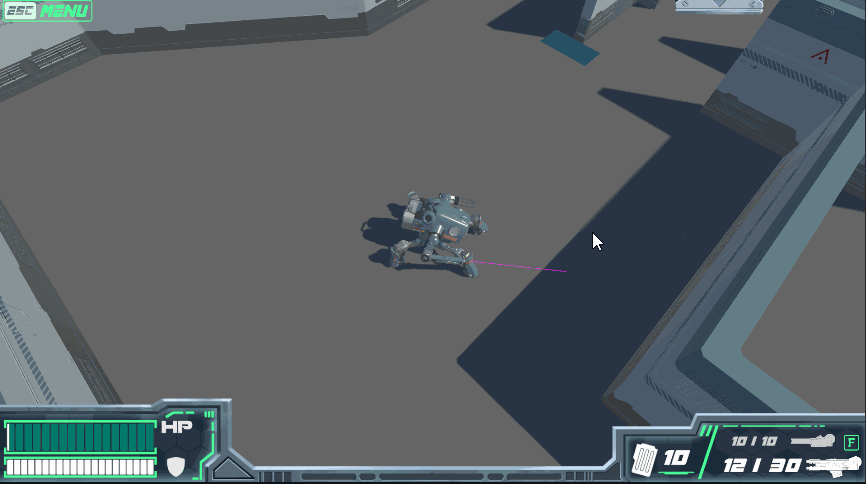
During development, I saw adding procedural animations as both an enjoyable addition to the game and a personal challenge. It seemed like a fun feature to incorporate, and I also viewed it as an opportunity to push my skills and explore new techniques in animation. The most challenging part was refining the leg movement animation, which required a lot of time for careful adjustments and fine-tuning. Because of these challenges, we decided to prioritize fine-tuning other features due to time constraints.
View whole script
private IEnumerator MoveLeg(int index, Vector3 targetPoint)
{
legsGrounded[index] = false;
Vector3 startPos = currentLegLocations[index];
float t = 0f;
while (t < 1f)
{
// Calculate the vertical offset based on time and step height
float yOffset = Mathf.Sin(t * Mathf.PI) * stepHeight;
// Interpolate horizontally towards the target position
t += Time.deltaTime / stepSpeed;
legs[index].position = Vector3.Lerp(startPos, targetPoint, t);
// Apply the vertical offset
legs[index].position += Vector3.up * yOffset;
yield return null;
}
legs[index].position = targetPoint;
currentLegLocations[index] = legs[index].position;
legsGrounded[index] = true;
}
Door System
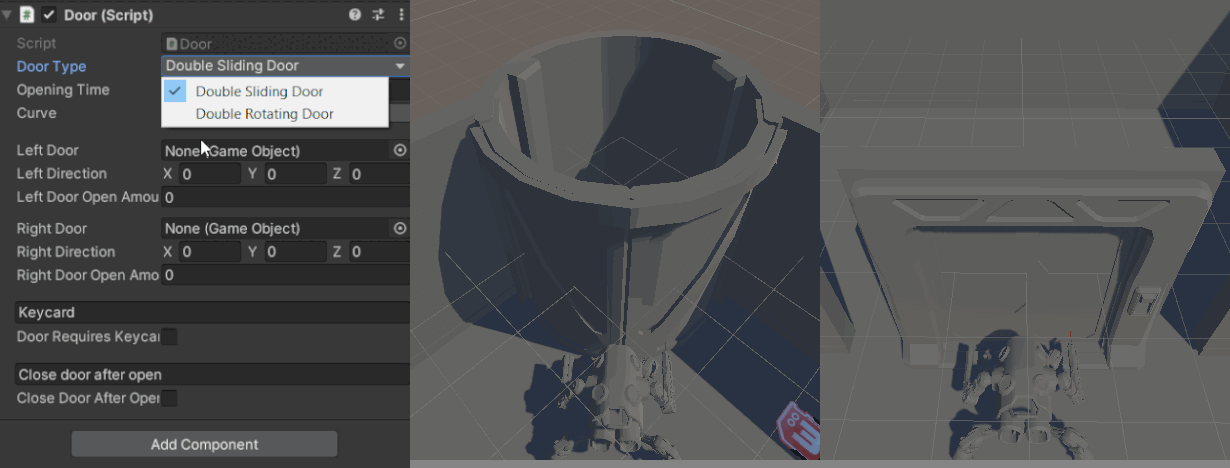
During development, we needed two types of doors: a rotating door and a sliding door. While they could have been assigned different classes, I opted to create a single script to handle both. To achieve this, I utilized a custom editor that serializes variables based on the selected door type. Although not the most performance-efficient solution, I prioritized ease of use for our artists.
View scriptOther features I also made
- Menu's
- Keycard system
- Player Movement
- Player Weapon
- UI implementation
- Audio system
View project on GitHub
What I learned from this project
In this project, I learned how to collaborate more effectively with both artists and developers. A key lesson I took away from this experience was the importance of adhering to the Single Responsibility Principle in my code. Throughout the project, I found myself frequently navigating through different scripts to locate specific implementations, as many of them attempted to handle multiple tasks simultaneously.